When you want a control to display little bitmaps next to its items you usually want them to come from your own images, or, if the items are files, you want the icons to be those that the system uses to represent the items file-type.
The easiest way to get a control to use pictures of your own creation is to create a bitmap 16 pixels high and as long as you need for the number of icons you will require (this is called IDB_ImageList).
Fill the background in an unused colour (Pink is the most popular RGB(255,0,255): tell the system not to draw pixels drawn in this colour (so pink areas will be transparent).
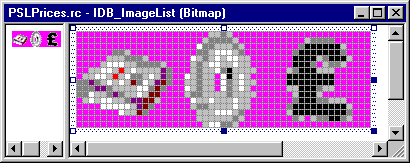
In the header file of your dialog class you need a CImageList:
CImageList ImageList;
In the dialog class OnInitDialog() method add the following two lines.
ImageList.Create(IDB_ImageList, 16, 1, RGB(255,0,255));
MyTreeControl.SetImageList(&ImageList,TVSIL_NORMAL);
If its not a tree control you'll have to look up the appropriate SetImageList Parameters:
MyListControl.SetImageList(&ImageList,LVSIL_SMALL);
So how do you get a control to put a File Item's Icon in a control?
Well, there may be a better way, but the following is reasonable:
void SetImage(const CString& Path) {
SHFILEINFO FileInfo;
HIMAGELIST HImageList=(HIMAGELIST)SHGetFileInfo(Path, FILE_ATTRIBUTE_NORMAL, &FileInfo, sizeof(FileInfo), SHGFI_USEFILEATTRIBUTES | SHGFI_SYSICONINDEX | SHGFI_SMALLICON);
CImageList* pImageList=CImageList::FromHandle(HImageList);
if(pImageList) MyTreeControl.SetImageList(pImageList,TVSIL_NORMAL);
MyTreeControl.SetImage(FileInfo.iIcon);
}
void SetFolderImage() {
SHFILEINFO FileInfo;
HIMAGELIST HImageList=(HIMAGELIST)SHGetFileInfo("", FILE_ATTRIBUTE_DIRECTORY, &FileInfo, sizeof(FileInfo), SHGFI_USEFILEATTRIBUTES | SHGFI_SYSICONINDEX | SHGFI_SMALLICON);
CImageList* pImageList=CImageList::FromHandle(HImageList);
if(pImageList) MyTreeControl.SetImageList(pImageList,TVSIL_NORMAL);
MyTreeControl.SetImage(FileInfo.iIcon);
}
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 'AS IS'
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.